EEPROM Library
EEPROM allows you to permanently store small amounts of data, which is very useful for saving settings, collecting small data sets, or any other use where you need to retain data even if the power is turned off.Download: EEPROM is included with Arduino
Hardware Requirements
All Teensy boards have EEPROM memory build inside the chip. No extra hardware is required. The demo program reads an analog voltage and stores it into EEPROM, so a trim pot was connected to analog input A0.
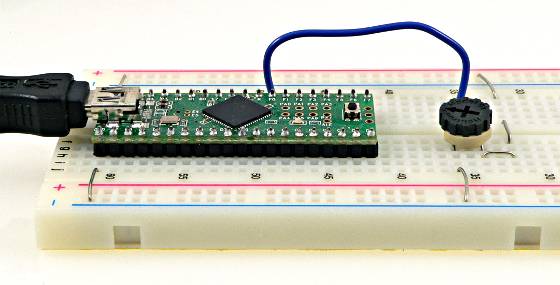
Each Teensy has a different amount of EEPROM memory available.
Board | EEPROM Size |
---|---|
Teensy 4.1 | 4284 bytes |
Teensy 4.0 | 1080 bytes |
Teensy 3.6 | 4096 bytes |
Teensy 3.5 | 4096 bytes |
Teensy 3.2 | 2048 bytes |
Teensy 3.1 | 2048 bytes |
Teensy 3.0 | 2048 bytes |
Teensy LC | 128 bytes |
Teensy++ 2.0 | 4096 bytes |
Teensy 2.0 | 1024 bytes |
Teensy++ 1.0 | 2048 bytes |
Teensy 1.0 | 512 bytes |
Teensy 3.6 can not write to EEPROM memory when running faster than 120 MHz. The EEPROM library will automatically reduce the processor's speed during the time EEPROM data is written. If using Serial1 or Serial2, communication may be disrupted due to baud rate changes. Other serial ports are not affected by the temporary speed change during EEPROM writing.
Basic Usage
EEPROM.read(address)Read a byte (0 to 255) from the EEPROM. Address is the location within the EEPROM.
EEPROM.write(address, data)Write a byte (0 to 255) to the EEPROM. Address is the location to store the byte, and data is the number to store.
Address can range from 0 to the EEPROM size minus 1. For a Teensy 2.0, Address can be 0 to 1023, for a total of 1024 unique bytes that can be stored in the EEPROM.Example Program
The examples can be opened from the File -> Examples -> EEPROM menu.The Write example was run while slowly turning the trim pot counter clockwise, where it wrote decreasing numbers into the EEPROM. Then the Read example was run, producing this output:
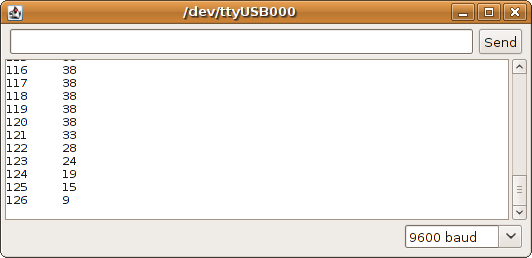
/* EEPROM Read * * Reads the value of each byte of the EEPROM and prints it * to the computer. */ #include <EEPROM.h> // start reading from the first byte (address 0) of the EEPROM int address = 0; byte value; void setup() { Serial.begin(9600); } void loop() { // read a byte from the current address of the EEPROM value = EEPROM.read(address); Serial.print(address); Serial.print("\t"); Serial.print(value, DEC); Serial.println(); // advance to the next address of the EEPROM address = address + 1; // there are only 512 bytes of EEPROM, from 0 to 511, so if we're // on address 512, wrap around to address 0 if (address == 512) address = 0; // Teensy 1.0 has 512 bytes // Teensy 2.0 has 1024 bytes // Teensy++ 1.0 has 2048 bytes // Teensy++ 2.0 has 4096 bytes delay(500); }
EEPROM Write Endurance
The EEPROM is specified with a write endurance of 100,000 cycles. Each time you write, the memory is stressed, and eventually it will become less reliable. It's guaranteed to work for at least 100,000 writes, and will very likely work for many more. Normally this limit is not an issue if you write to the EEPROM infrequently.The 100,000 writes apply to each address separately. For example, if you write to address 0 many times, only that address has been stressed. Address 1, 2, 3, etc would not be stressed by writing to address 0. If you do write data quickly, you can prolong the life of the EEPROM by cycling through all addresses in sequence.
Reading does not stress the EEPROM. Only writes count for the write endurance.