DS1307RTC Library
DS1307RTC allows you to access real time clock (RTC) chips compatible with the DS1307. It is intended to be used with the Time library.
Download: |
Included with the Teensyduino Installer Latest Developments on Github |
Hardware Requirements
DS1307RTC works with the DS1307, DS1337 and DS3231 real time clock chips.
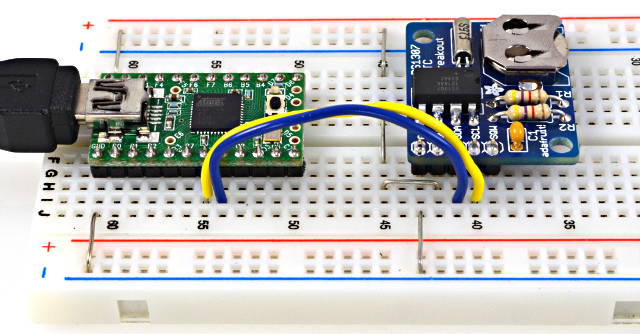
The DS1307 chip only works with 5 volt power. The DS1337 and DS3231 can use 3.3 or 5 volts.
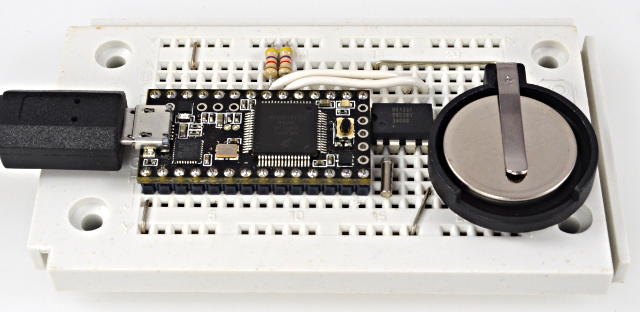
Teensy 3.0 has a built-in RTC, so the DS1337 offers little benefit. However, the DS3231 includes a temperature compensated crystal, so it is recommended for applications requiring high accuracy.
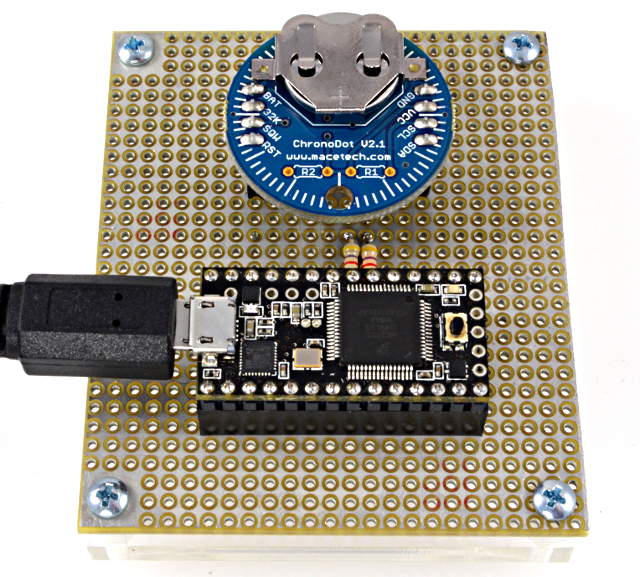
The ChronoDot board provides the DS3231 chip in an easy-to-use package. For use with Teensy 3.0, you must add pullup resistors for the SDA and SCL signals.
Basic Usage
RTC.get();Reads the current date & time as a 32 bit "time_t" number. Zero is returned if the DS1307 is not running or does not respond.
RTC.set(t);Sets the date & time, using a 32 bit "time_t" number. Returns true for success, or false if any error occurs.
RTC.read(tm);Read the current date & time as TimeElements variable. See the Time library for TimeElements details. Returns true on success, or false if the time could not be read.
RTC.write(tm);Sets the date & time, using a TimeElements variable. Returns true for
RTC.chipPresent();Returns true if a DS1307 compatible chip was present after using the 4 functions. If an error occurs, this can be used to distinguish between a DS1307 that is not running vs no chip connected at all.
Example Program
DS1307RTC includes 2 examples, to read or set the time.You can open this example from File > Examples > DS1307RTC > ReadTest.
#include <DS1307RTC.h> #include <Time.h> #include <Wire.h> void setup() { Serial.begin(9600); while (!Serial) ; // wait for serial delay(200); Serial.println("DS1307RTC Read Test"); Serial.println("-------------------"); } void loop() { tmElements_t tm; if (RTC.read(tm)) { Serial.print("Ok, Time = "); print2digits(tm.Hour); Serial.write(':'); print2digits(tm.Minute); Serial.write(':'); print2digits(tm.Second); Serial.print(", Date (D/M/Y) = "); Serial.print(tm.Day); Serial.write('/'); Serial.print(tm.Month); Serial.write('/'); Serial.print(tmYearToCalendar(tm.Year)); Serial.println(); } else { if (RTC.chipPresent()) { Serial.println("The DS1307 is stopped. Please run the SetTime"); Serial.println("example to initialize the time and begin running."); Serial.println(); } else { Serial.println("DS1307 read error! Please check the circuitry."); Serial.println(); } delay(9000); } delay(1000); } void print2digits(int number) { if (number >= 0 && number < 10) { Serial.write('0'); } Serial.print(number); }