FastLED Library
The FastLED library, by Daniel Garcia, allows you to many types of LED strips.
Download: |
Included with the Teensyduino Installer Latest Developments on Github |
Hardware Requirements
FastLED works with all Teensy models.
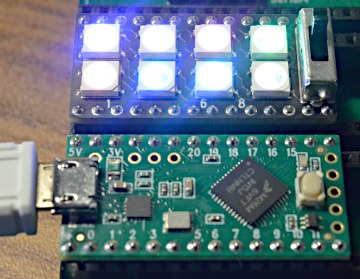
Example Program
#include <FastLED.h> #define DATA_PIN 1 #define NUM_LEDS 8 CRGB leds[NUM_LEDS]; elapsedMillis tic; void setup() { Serial.begin(115200); Serial.println("FastLed Test"); FastLED.addLeds<WS2812, DATA_PIN, GBR>(leds, NUM_LEDS); FastLED.setBrightness(50); for (int i=0; i < NUM_LEDS; i++) { leds[i] = CRGB::Black; } } void loop() { if (tic >= 333) { /* Shift prior colors down all the LEDs */ for (int i=NUM_LEDS-1; i > 0; i--) { leds[i] = leds[i - 1]; } /* Turn the first LED on with a random color */ uint8_t red = random8(); uint8_t green = random8(); uint8_t blue = random8(); Serial.printf("R=%3d G=%3d B=%3d\n", red, green, blue); leds[0].setRGB(red, green, blue); FastLED.show(); tic = 0; } }
High Performance Non-Blocking Drivers
FastLED can use the OctoWS2811 and WS2812Serial libraries as LED device drivers, giving you higher performance together with FastLED's many special color rendering features.To see the proper syntax, open these examples.
- File > Examples > WS2812Serial > FastLED_Cylon
- File > Examples > OctoWS2811 > BasicTest_FastLED